iOS Security Best Practices: Protecting User Data in Your Apps
Boost your iOS app's security with tips on data storage, network protocols, user authentication, and incident handling. Explore now at Tectoks.com!
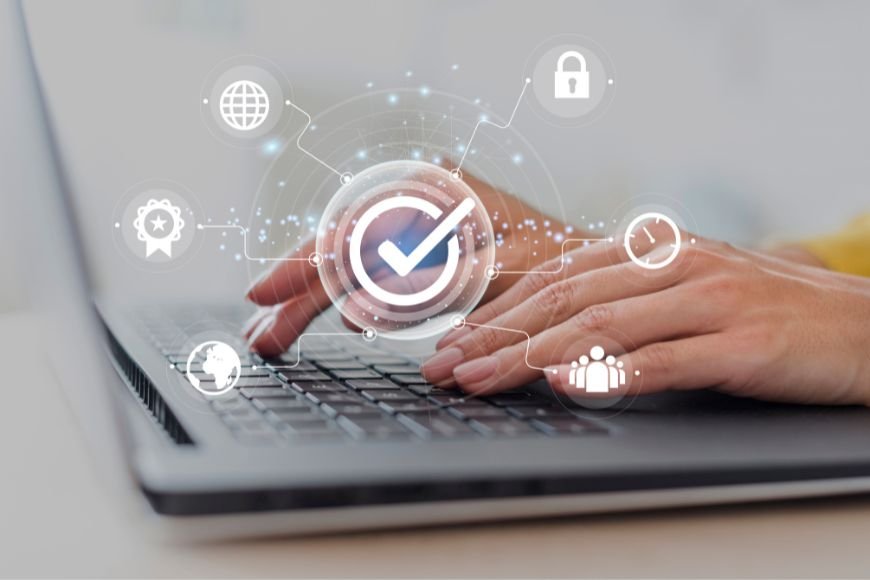
Table of content
-
Introduction
-
Secure Data Storage
-
Network Communication Security
-
User Authentication and Authorization
-
Secure Code Practices
-
Handling Security Incidents
-
Conclusion
Introduction
iOS is one of the most popular and secure mobile operating systems in the world. It offers a range of features and technologies to protect the privacy and integrity of user data and app functionality. However, as an iOS developer, you also have a responsibility to follow the best practices and guidelines to ensure that your app is secure and compliant with the latest standards and regulations. One of these aspects is iOS device management, which allows you to control and monitor the devices that run your app, and enforce policies and restrictions to prevent unauthorized or harmful use. In this blog post, we will cover some of the most important aspects of iOS security, such as data storage, network communication, user authentication, code practices, and incident handling. By following these best practices, you can improve the security of your app and provide a better user experience.
Secure Data Storage
One of the key aspects of iOS security is how you store and manage the data that your app uses or generates. Data can be stored in various locations, such as the device’s memory, file system, or cloud services. Depending on the type and sensitivity of the data, you need to apply different levels of protection and encryption to prevent unauthorized access or modification.
Utilizing Keychain Services
Keychain Services is a secure and encrypted storage system that allows you to store small pieces of sensitive data, such as passwords, tokens, certificates, or keys. Keychain Services is integrated with the device’s hardware and software, and provides a high level of security and convenience. You can access the keychain data using the Keychain API, which handles the encryption and decryption for you. You can also specify the access level and attributes for each keychain item, such as whether it requires user authentication, biometric verification, or device passcode. Keychain Services is recommended for storing any data that is critical for your app’s functionality or security, such as credentials, encryption keys, or API tokens.
File Encryption Techniques
File encryption is a technique that transforms the data in a file into an unreadable format using a secret key. Only the authorized parties who have the key can decrypt and access the original data. File encryption can be applied to any data that is stored in the device’s file system, such as documents, images, videos, or databases. File encryption can protect the data from being accessed or tampered with by malicious actors, such as hackers, malware, or thieves.
There are two primary types: symmetric and asymmetric encryption.
Symmetric encryption uses a single key for both encryption and decryption, while asymmetric encryption employs a public-private key pair. Examples include AES for symmetric encryption and RSA for asymmetric encryption.
Utilize iOS frameworks like CryptoKit or third-party libraries like CommonCrypto for implementation, or leverage the Data Protection API for automatic encryption based on device lock state.
Implementing Data Protection Classes
Data Protection Classes are a feature that allows you to specify the level of protection and accessibility for each file or keychain item. Data Protection Classes are based on the device’s lock state, which is determined by whether the user has unlocked the device with a passcode, Touch ID, or Face ID. Data Protection Classes can help you balance the security and usability of your app, by allowing you to choose the most appropriate level of protection for each type of data. There are four Data Protection Classes that you can use in iOS:
-
Complete Protection: Data accessible only when device is unlocked. Default for sensitive info.
-
Protected Unless Open: Accessible when device is unlocked or file is open.
-
Protected Until First User Authentication: Accessible after device's first unlock.
-
No Protection: Accessible at any time, even when device is locked.
You can set the Data Protection Class for each file or keychain item using the NSFileProtectionKey attribute or the kSecAttrAccessible attribute, respectively. You can also use the FileProvider framework to implement Data Protection Classes for files that are stored in external locations, such as iCloud Drive or third-party cloud services.
Network Communication Security
Another key aspect of iOS security is how you communicate and exchange data with external servers or services over the network. Network communication can expose your app and your users to various threats, such as eavesdropping, interception, modification, or impersonation. To prevent these threats, you need to apply different levels of security and encryption to your network requests and responses.
Enforcing HTTPS for Network Requests
HTTPS is a protocol that encrypts the data that is transmitted between the client and the server using SSL/TLS certificates. HTTPS can protect the data from being read or modified by anyone who is not authorized to access it. Enforcing HTTPS ensures safeguarding against unauthorized access or modification. It verifies server identity and authenticity through certificate validation. Recommended for sensitive data like logins or payments, HTTPS is implemented via URLSession API, simplifying SSL/TLS handshake and validation. Third-party libraries like Alamofire or AFNetworking streamline network communication. Additionally, NSAppTransportSecurity (ATS) settings, discussed later, restrict network communication to HTTPS exclusively.
Implementing Certificate Pinning
Implementing certificate pinning involves specifying the exact certificate or public key expected from the server, enhancing network communication security. This prevents man-in-the-middle attacks where certificates are replaced with fraudulent ones, and safeguards against compromised certificate authorities. URLSession API allows customization of certificate validation via URLSessionDelegate protocol, while third-party libraries like TrustKit or PinningSSL streamline the process. Additionally, NSAppTransportSecurity (ATS) settings, discussed later, enable specifying expected server certificates or public keys.
Using App Transport Security (ATS) Settings
App Transport Security (ATS) in iOS upholds network communication security standards by mandating HTTPS with robust encryption and valid certificates. Customizable ATS settings in the Info.plist file enable tailoring network security policies. Key settings include:
-
NSAllowsArbitraryLoads: Disables ATS for all network requests, not recommended except for valid legacy server compatibility.
-
NSExceptionDomains: Specifies domains exempt from ATS requirements, useful for domains without HTTPS support or weak encryption.
-
NSPinnedDomains: Specifies domains requiring certificate pinning, ensuring expected server certificates or public keys, critical for app security like authentication or payments.
Utilize these settings judiciously to maintain app security and compliance.
User Authentication and Authorization
User authentication and authorization are the processes of verifying the identity and permissions of the users who access your app or its features. One of the methods to enhance user authentication and authorization is two factor authentication iphone, which requires both your Apple ID password and a six-digit verification code to sign in with your Apple ID . This can protect your app and your users from unauthorized or malicious access or actions. User authentication and authorization can also provide a personalized and customized user experience, by allowing you to tailor the app’s content and functionality based on the user’s profile and preferences.
Secure Password Handling
Passwords are vital for user authentication but can pose security risks if mishandled. Follow best practices:
-
Generating Strong and Random Passwords
Create passwords with ample length, complexity, and randomness to thwart brute-force or dictionary attacks. Utilize Password AutoFill, third-party services like 1Password, or the Security framework.
-
Storing and Transmitting Passwords Securely
Safeguard passwords using techniques like hashing, salting, and bcrypt. Employ Keychain Services, File Encryption, Data Protection Classes, HTTPS, Certificate Pinning, and ATS for secure storage and transmission. Use CryptoKit or third-party libraries for implementation.
Implementing Two-Factor Authentication
Implementing Two-Factor Authentication (2FA) adds an extra layer of security to user authentication by requiring two forms of evidence for identity verification. Typically, this involves something the user knows (like a password) and something they have (like a phone). 2FA mitigates brute-force and phishing attacks.
You can implement 2FA using:
-
The LocalAuthentication framework for device biometrics (Touch ID/Face ID).
-
Third-party services such as Firebase Authentication or Auth0, offering options like SMS, email, or push notifications.
-
The Web Authentication API, utilizing hardware security keys like YubiKey or Titan.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a method for defining and enforcing user permissions and privileges within an app. It enables assigning different roles (e.g., admin, moderator, user) to regulate access and functionality. RBAC aids in compliance with data regulations like GDPR, HIPAA, or COPPA.
Implementation options include:
-
Utilizing the CloudKit framework for role and record management.
-
Leveraging third-party services like Firebase Realtime Database or Parse for authentication, authorization, and encryption.
-
Employing the Security framework for creating and managing access control lists (ACLs) and policies for app files or keychain items.
Secure Code Practices
Secure code practices are the principles and guidelines that you should follow to write and maintain the code for your app. Secure code practices can help you prevent and mitigate the risks and vulnerabilities that can compromise the security and functionality of your app, such as bugs, errors, or exploits. Secure code practices can also help you improve the quality and performance of your app, by making your code more readable, maintainable, and testable.
Avoiding Hardcoding Sensitive Information
Avoiding hardcoding sensitive information involves refraining from embedding confidential data directly into your app's source code, such as passwords, keys, tokens, or URLs. This practice exposes the data to potential unauthorized access or reverse-engineering. Additionally, updating or revoking such information becomes cumbersome, requiring app recompilation and redeployment.
Instead, opt for storing sensitive data in secure and encrypted locations like Keychain Services, File Encryption, or Data Protection Classes. Utilize the Secure Enclave, a hardware-based security feature, for extremely sensitive data like cryptographic keys or biometric data. Alternatively, employ third-party services like Firebase Remote Config or AWS Parameter Store for secure and dynamic configuration options.
Regularly Updating Third-Party Libraries
Third-party libraries are external code or resources that you can use in your app to provide additional features or functionality, such as networking, analytics, or UI components.Regularly updating third-party libraries is crucial for maintaining app security and functionality. While these libraries offer convenient features, they can also pose security risks if left outdated.
To ensure the safety of your app:
-
Regularly check for updates and patches from official sources or repositories.
-
Review changelogs and documentation to understand changes and potential compatibility issues.
-
Test updated libraries in your app to verify expected functionality and security integrity.
Performing Code Reviews and Static Analysis
Code reviews and static analysis are techniques that allow you to inspect and evaluate the quality and security of your code, by using manual or automated tools or processes. Performing code reviews and static analysis is essential for assessing the quality and security of your codebase. These techniques help identify and rectify bugs, errors, and vulnerabilities before they impact your app or users. Additionally, they enhance code readability, maintainability, and performance by adhering to coding standards and best practices.
To conduct thorough evaluations:
-
Utilize Xcode's built-in tools or third-party solutions like SwiftLint, SonarQube, or Codebeat.
-
Adhere to guidelines and checklists provided by reputable sources such as Apple Developer, OWASP, or Swift.org.
-
Encourage peer involvement to gain diverse feedback and insights during the review process.
Handling Security Incidents
Security incidents are events or situations that compromise or threaten the security or functionality of your app or your users, such as data breaches, cyberattacks, or system failures. Security incidents can cause serious consequences and damages, such as loss of data, reputation, or revenue, legal liabilities, or user dissatisfaction. To prevent or mitigate these consequences and damages, you need to have a plan and a procedure to handle the security incidents that may occur in your app.
Monitoring and Logging for Suspicious Activities
Monitoring and logging are crucial techniques for collecting and analyzing app-related data, including network requests, user actions, and system errors. They aid in detecting and responding to security incidents such as unauthorized access or data leakage. Moreover, they facilitate investigation and troubleshooting by providing evidence and details.
To implement effective monitoring and logging:
-
Utilize built-in solutions like the OSLog framework or third-party services such as Firebase Crashlytics, Sentry, or Bugsnag.
-
Configure settings for data collection frequency, format, and analysis level.
-
Regularly review and audit monitoring and logging data to address security issues promptly.
Incident Response Plan and Procedures
An incident response plan is a document that defines and describes the goals, roles, and responsibilities of the people and processes that are involved in handling the security incidents that may occur in your app. It ensures preparedness and coordination, guiding actions and resource allocation based on industry best practices. Additionally, it aids in evaluating and enhancing incident response effectiveness and efficiency through defined metrics
To develop an effective incident response plan:
-
Utilize templates and examples from reputable sources such as Apple Developer, NIST, or SANS.
-
Regularly update and test the plan to align with evolving security threats.
-
Provide training and education to your team to ensure readiness in handling security incidents.
Communication Strategies with Users in Case of Breach
Communication strategies with users during a security incident involve methods and channels used to inform and engage users affected by events like data breaches or cyberattacks. These strategies aim to provide accurate information, reassure users, and restore trust.
To implement effective communication strategies:
-
Utilize suitable channels such as email, push notifications, or in-app messages.
-
Follow guidelines and best practices from reputable sources like Apple Developer, FTC, or GDPR.
-
Be transparent, honest, and take responsibility for the incident, providing users with appropriate actions like password changes or app updates.
Conclusion
In conclusion, ensuring the security of your iOS app is paramount to protecting user data and maintaining user trust. By adhering to best practices across various aspects of iOS security, such as secure data storage, network communication, user authentication, and incident handling, you can significantly mitigate risks and enhance the overall security posture of your app. Regularly updating third-party libraries, performing code reviews, and having an incident response plan in place are essential steps towards maintaining app security. Additionally, effective communication strategies with users during security incidents are crucial for transparency and trust-building. By prioritizing security at every stage of app development and implementation, you not only safeguard user data but also provide a better and more secure user experience.
What's Your Reaction?
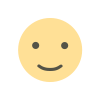
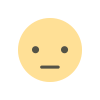
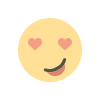
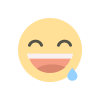
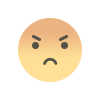
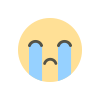
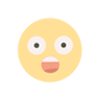